to focus on your job, but...

advanced filters in your application with Refine.
for your Laravel and Rails applications.
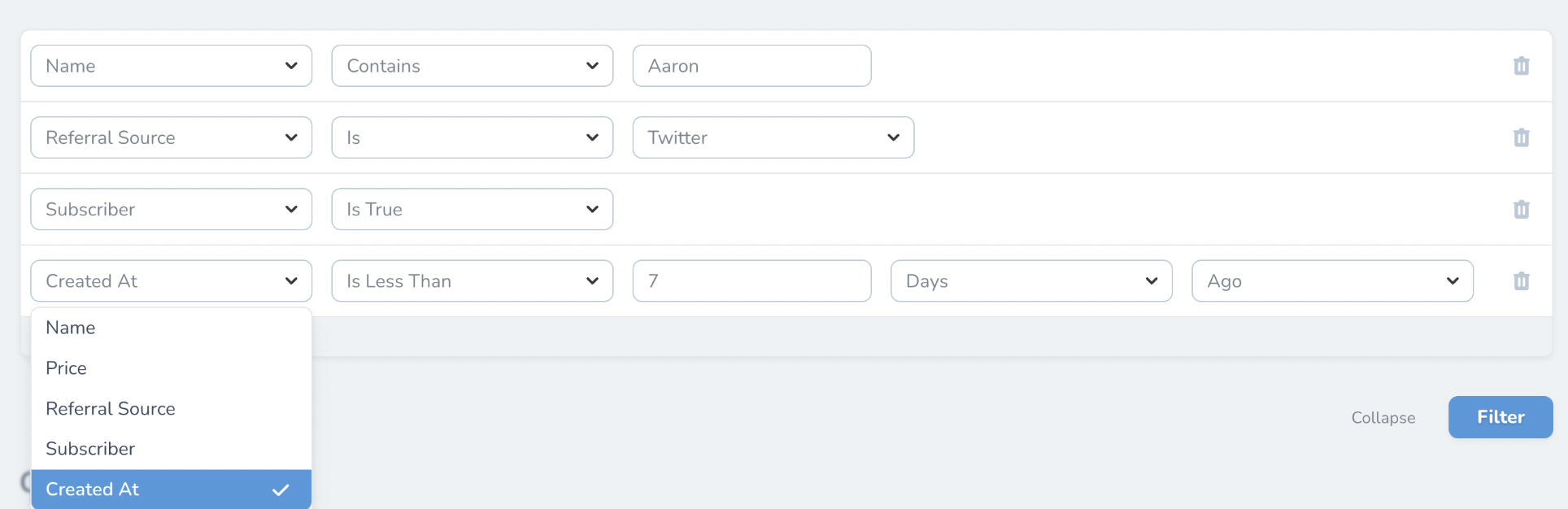
bundle install
or composer require
.
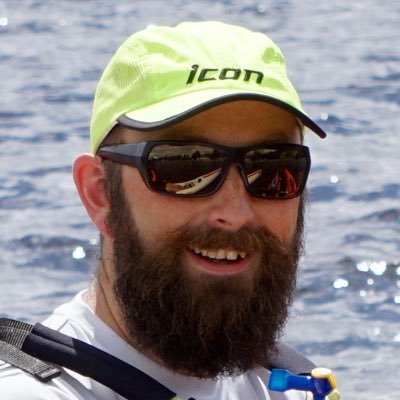
Before Refine I was spending at least an hour a day searching for teams with certain subscription states and pupil numbers. Now it takes me seconds.
Already convinced? Get started today.
How does it work?
After you've added Refine using bundle
or composer
you create a new
Filter class that extends the class that ships with Refine.
Define an initial query. Refine builds up the final query based on the initial query and the user's input.
class UserFilter < Hammerstone::Refine::Filter def initial_query User.all endend
class UserFilter extends Filter{ public function initialQuery() { return User::query(); }}
Your users can filter data based on a set of conditions that you configure. Each condition corresponds to an attribute on your model.
You tell Refine the name of the column and its type, and we'll handle the rest.
class UserFilter < Hammerstone::Refine::Filter def initial_query User.all end def conditions [ TextCondition.new("email"), NumericCondition.new("nps_score") ] endend
class UserFilter extends Filter{ public function initialQuery() { return User::query(); } public function conditions() { return [ TextCondition::make('email'), NumericCondition::make('nps_score') ]; }}
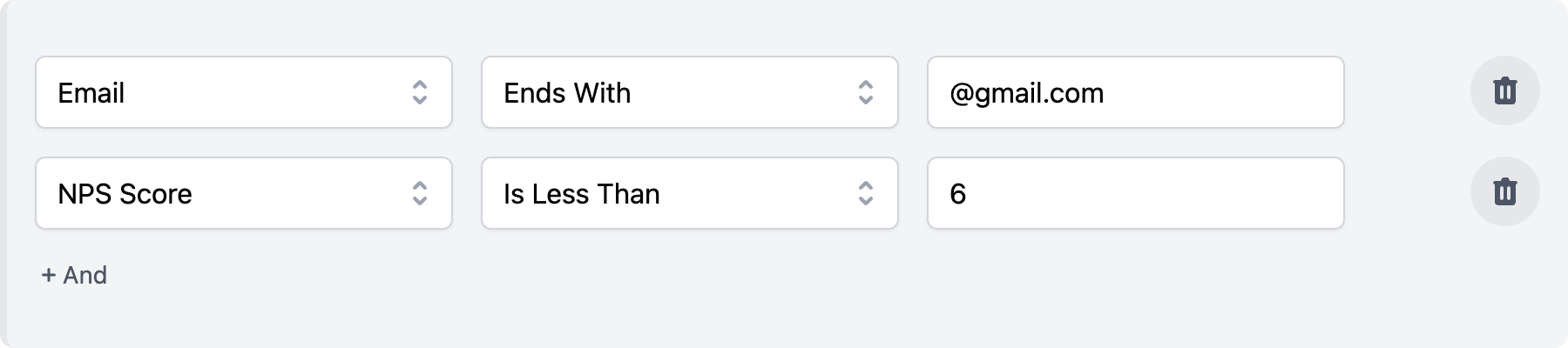
Refine gives you a set of prebuilt conditions that covers most use cases:
- Text — starts with, contains, ends with, equals; we do it all.
- Date, datetime, and timestamps — don't worry, we handle the timezones.
- Boolean — true/false columns, with optional support for nulls.
- Numeric — greater, less, equal, between; everything you'd expect.
- Option — pick one or more from a defined set.
All of these can be combined with and/or groupings. And if those don't meet your needs, you can add your own custom conditions!
What about relationships?
User
has an Address
with a state_id
that you want to include in your filter, you can add address.state_id
as a condition.
class UsersFilter < Hammerstone::Refine::Filter def initial_query User.all end def conditions [ TextCondition.new("email"), NumericCondition.new("nps_score") OptionCondition.new("address.state_id") .with_display("State") .with_options(State.all.pluck(:name, :id)) ] endend
class UserFilter extends Filter{ public function initialQuery() { return User::query(); } public function conditions() { return [ TextCondition::make('email'), NumericCondition::make('nps_score'), OptionCondition::make('address.state_id') ->display('State') ->options(function() { return State::query()->pluck('name', 'id'); }) ]; }}
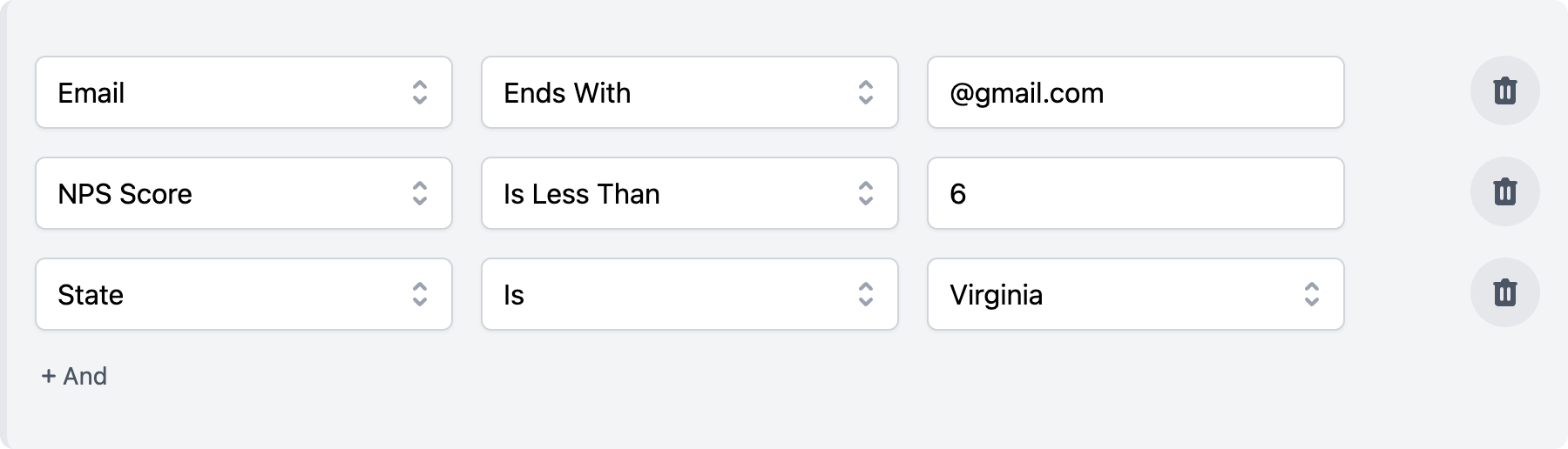